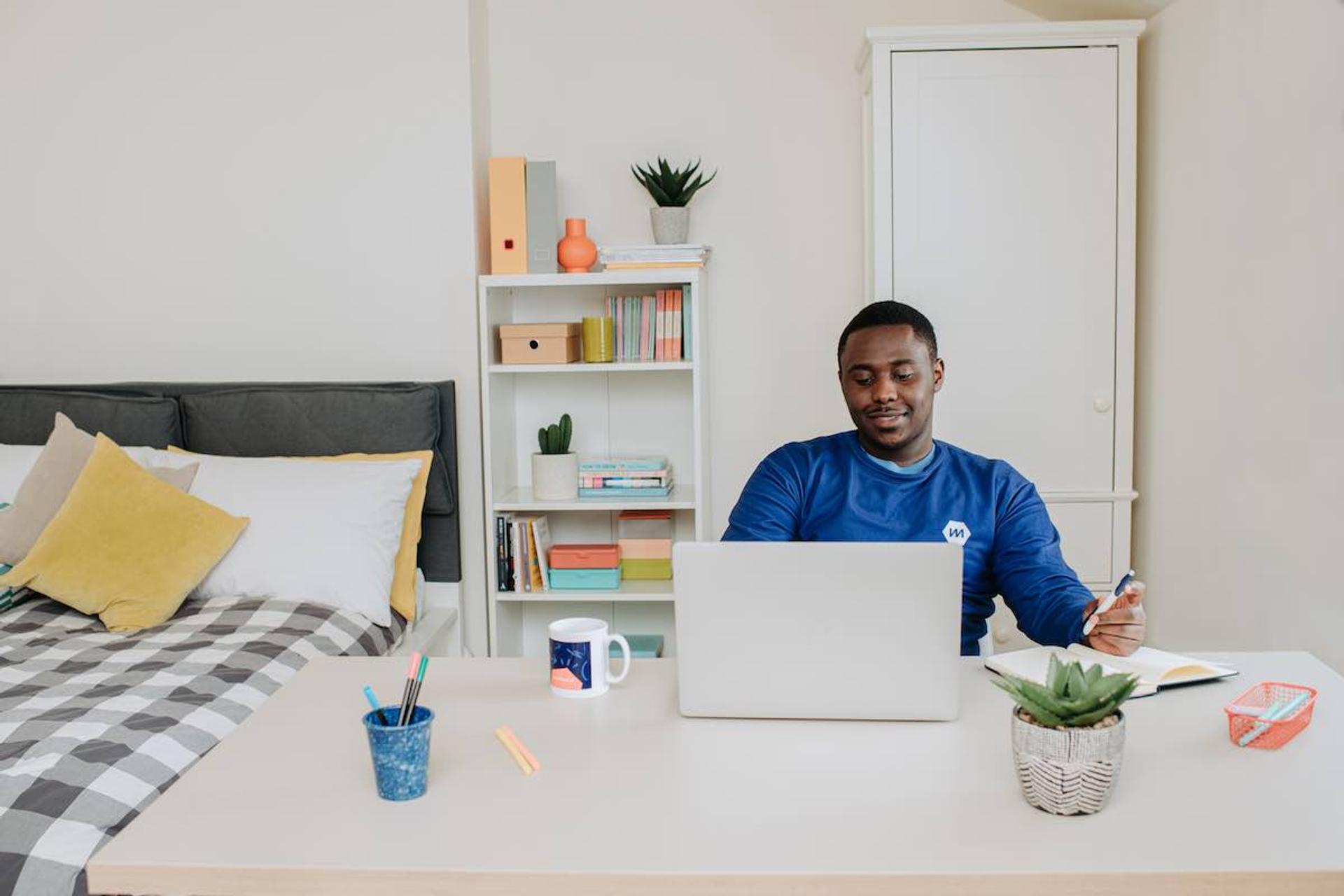
Few programming languages have shaped the digital world like JavaScript (JS). An estimated 98% of websites use JavaScript. But what is JavaScript, and why is it everywhere on the internet?
Many web developers and programmers start off learning hypertext markup language (HTML), cascading style sheets (CSS), and JavaScript. If you’re interested in building websites, you’ll need to know each of these languages. You’ll also need to understand how they come together to create a functional website.
In this post, we’re focusing on JavaScript. We’ll cover everything you need to know—from what JavaScript is to how developers use it to build dynamic and interactive websites.
What is JavaScript?
JavaScript is a programming language. It’s one of the three—including HTML and CSS—that shape the modern web and are all necessary for web development. Before you can understand JavaScript—you need to know how it works with HTML and CSS.
HTML code makes up the building blocks of the web. It structures web pages and tells a browser what to display. You can use this code to create page titles, headings, tables, hyperlinks, images, and other HTML elements.
CSS tells the browser how to display the elements on a web page. For example, to add styling to your headings, you’ll need CSS to set the font size, colors, and so on. CSS identifies the HTML code you want and how to style it.
JavaScript builds on these two programming languages to add interactive behavior and dynamic web pages. Take an email sign-up form as an example. HTML creates the structure of the form and button. CSS styles it. JavaScript makes the form and button work. It can tell the browser where to send the data and what to do after someone clicks the button, like taking them to a thank you confirmation page.
JavaScript does much more to enable user interaction than create sign-up forms. Developers use this language to add dynamic content and interactive elements to the front end—or client side—of the website. They can also use JavaScript to build back-end functions that users don’t directly access, such as data retrieval and user authentication.
Websites created using only HTML and CSS allow you to view content, but you can’t interact directly with any elements on the page. JavaScript makes websites dynamic and interactive. For example, you can add charts that update in real-time, rotating image carousels, interactive maps, and more.
History of JavaScript
JavaScript’s central role in web development dates back to the beginning of the internet. The earliest websites were static. You only saw what was loaded onto the HTML page, and couldn’t interact with it. Websites were like printed posters that you viewed and accessed online.
The browser Netscape wanted to create a new language to make web pages more dynamic. In 1995, Brendan Eich, a computer programmer at Netscape, created JavaScript. He based JavaScript’s language and rules on the existing programming languages Java and Scheme.
Not long after, the browser wars happened. Several major web browsers—Internet Explorer, Netscape, Firefox, Google Chrome, Safari, and others—competed to be the top browser for internet users.
If you aren’t familiar with Netscape, it’s because it didn’t last long. It was replaced by competitors like Google Chrome, which dominates the market, with 65% of users opting for it(opens new window).
JavaScript grew in popularity and became the standard for all web pages and major browsers.
Why should you learn JavaScript?
All types of companies, from big brands to growing startups, need skilled JS developers for front-end development and client-side scripting. Because of its high demand, learning JS can open up thousands of job opportunities in various industries. Many of these careers offer lucrative salaries. For example, the average base Software Engineer salary in the United States is $116,962(opens new window).
With JS knowledge and experience, you can also pursue a career in front-end or back-end development, full stack development, or game development.
How JavaScript works
When a user opens a webpage, their browser runs the JavaScript code that comes with it. JavaScript adds behavior, from making forms work (taking form input and making buttons functional) to creating in-browser games.
Modern web browsers have internal JavaScript engines, which run the code and help render the page. But how do you get your JS code onto a website?
Adding JavaScript to a website
The most common way to add JS to a website is to create a separate .js file that you’ll refer to within your HTML content. Typically, you’ll instruct this in either the <head> tag to load ASAP or the <body> tag if you want it to load after the rest of the HTML above it.
You’ll insert the separate .js file into your HTML with this snippet of code:
<script src=[folder path to the file]></script>
This is the preferred method you’ll see on the internet, but it’s not the only one. You can also add regular JavaScript code to HTML with that same <script> tag. All you need to do is include the code you want to run between the opening and closing script tags.
JavaScript frameworks
Developers also use JavaScript with frameworks to create sites efficiently. Frameworks provide a foundation and tools for building websites and applications. Many websites have similar features, so frameworks use this to their advantage by compiling a library that includes common pre-written components, such as:
- Scenarios
- Structures
- Code
JavaScript developers can borrow and customize these bits of code to build their own web and mobile applications without starting from scratch. It’s like getting a kit for building a house. The framework is the kit. Common JS frameworks include:
Instead of trying to start from scratch, you have a foundation ready to go. You also don’t need to memorize complicated blocks of code to create sophisticated JS functionality. Frameworks have libraries of pre-written code that you can repurpose for your development projects.
With frameworks like Meta’s React, developers build entire websites with JavaScript.
What can you create with JavaScript?
Websites
As we’ve discussed, websites and web applications are the most popular way developers use JavaScript. Most web developers build websites with JavaScript and frameworks like Node.js and React. If you want to become a Website or Front-end Developer, it’s a good idea to practice working with one or two JS frameworks.
Mobile apps
You can also create full mobile apps that work natively on Android and iOS with frameworks like React Native(opens new window). While the React framework helps developers build websites, React Native is designed for mobile apps.
You can also build JavaScript mobile applications by leveraging two lesser-known platforms: Cordova(opens new window) and Ionic(opens new window). Apps developed with these tools behave just like any other native mobile app would. But they don’t require developers to learn a new language outside JavaScript to create a mobile app.
These mobile JavaScript code libraries also integrate into Node, React, Vue, and other JS frameworks.
Desktop software
Developers can use tools like Electron to create a web page that acts like a fully independent piece of software. The creators of Discord built their platform from the ground up with Electron, and it now boasts over 150 million users every month.
Web servers and APIs
With Node.js or Express.js, you can create web servers, APIs, and a complete website back end. These frameworks give you a JavaScript runtime environment that runs outside a browser. A runtime environment is the software infrastructure that provides everything your code needs to run.
Google tags (gtag.js) is a real-world example of JavaScript working together with APIs and frameworks. If you aren’t familiar with Google Tag Manager, you can use it to measure and track behavior on your site.
First, you need to add the tag to your site. It tracks events like clicks, purchases, page loading, and more. Then, you can view your site data in Analytics and other Google products. GTM uses JavaScript. You're writing JS code when you edit your GTM configurations, although you may not realize it. You can also customize your tracking with some JavaScript knowledge.
Examples of JavaScript
Plenty of companies and products rely on JavaScript every day. Some developers, like the creators of Discord, use JavaScript as the only programming language. Others combine Javascript with another server-side language, like Python.
Here are a few examples:
Facebook and Instagram
Facebook’s web version and mobile app developers used React and React Native, respectively.Facebook’s parent company created the React frameworks. Instagram, the other social media company under Meta’s umbrella, also uses JavaScript for its web and mobile app.
Netflix
The original streaming service used React to create the web version of its platform. You can check out the Netflix team’s detailed blog post(opens new window) that shares why they chose React and how they use it.
Uber
The ride-sharing platform uses Node.js on the server side to process ride requests and match users with drivers. Uber also uses JavaScript interactive elements on the client side, such as interactive maps and trip details.
JavaScript FAQ
What is JavaScript used for?
Web developers insert JavaScript to transform static web pages into dynamic and interactive ones. This language can add interactive features on the client side and server side. For example, developers use JavaScript to enhance the user interface with dropdown menus, animations, and other dynamic features.
Is it hard to learn Javascript code?
If you’re wondering if it’s hard to learn coding with JavaScript, don’t worry. Developers widely regard JavaScript as one of the easiest programming languages to learn, so it’s a great way to get your feet wet.
Learning JavaScript can also prepare you to learn more challenging programming languages like C++ and Ruby.
Do you need to know another programming language to learn JavaScript?
Most JavaScript developers recommend that beginners learn two other languages: HTML and CSS. These languages serve as the building blocks for all web pages. Most people can master them in a few days, so they’re a good start to learning basic programming practices.
Once you know how to create a static page, you can learn how to add JavaScript code to insert interactive elements and dynamic behavior.
Do you need to be good at math to learn JavaScript?
JavaScript only requires basic knowledge of arithmetic. For instance, you may need to use addition and subtraction to calculate the total cost of items in a virtual shopping cart or update counters.
While Web Developers don’t need advanced math skills for JavaScript implementation, they need the ability to think logically. You can practice this skill by completing JavaScript coding challenges.
Are Java and JavaScript the same?
Java and JavaScript sound similar but have different programming languages with distinct purposes. Developers use JavaScript scripting language to create dynamic and interactive elements. This code gets interpreted by popular web browsers when users open web pages.
By contrast, Java has a broader range of uses. Developers use this versatile programming language to create games, mobile applications, servers, and other web technologies. After writing the code, they compile—or translate—it into bytecode so computers can understand it.
What are JavaScript frameworks?
A JavaScript framework refers to collections of ready-made JavaScript code libraries. Web Developers can use this pre-written, customizable code to streamline everyday tasks and functions. For instance, they can use JavaScript frameworks like React to build user interfaces and create complex animations without writing code from scratch.
How to learn JavaScript
Learning JavaScript can take between six to nine months. If you have no prior coding experience, this process may take longer. Most developers will recommend that you learn HTML and CSS first.
Luckily, because JS is everywhere on the internet, there are numerous resources to help you. Whether you want to know the basics or polish and hone your craft, these tips and resources can help you get started.
Start with simple syntax
Learning a programming language is a lot like learning a new language. You must learn the syntax—the rules, structure, and meaning of the language—before you can practice and become fluent.
First, cover the essential components of JavaScript code–variables and functions. Then, you can get into more complex topics like decision-making and arrays.
Mozilla’s MDN(opens new window) is a free resource that many developers use every day. It has guides on HTML, CSS, JavaScript, and more. You’ll also find examples of JS code with explanations of how you can use it. If you forget and Google the code snippet you need, MDN will most likely appear at the top of search results with the answer.
Download a code editor
You can write code in any text editor, like Word, Notepad, or Google Docs. But these tools aren’t designed with writing code in mind.
Instead, download and practice using a code editor like Atom or Visual Code Studio. These programs resemble text editors, but they’re designed for programming. They have features that help you write better code faster.
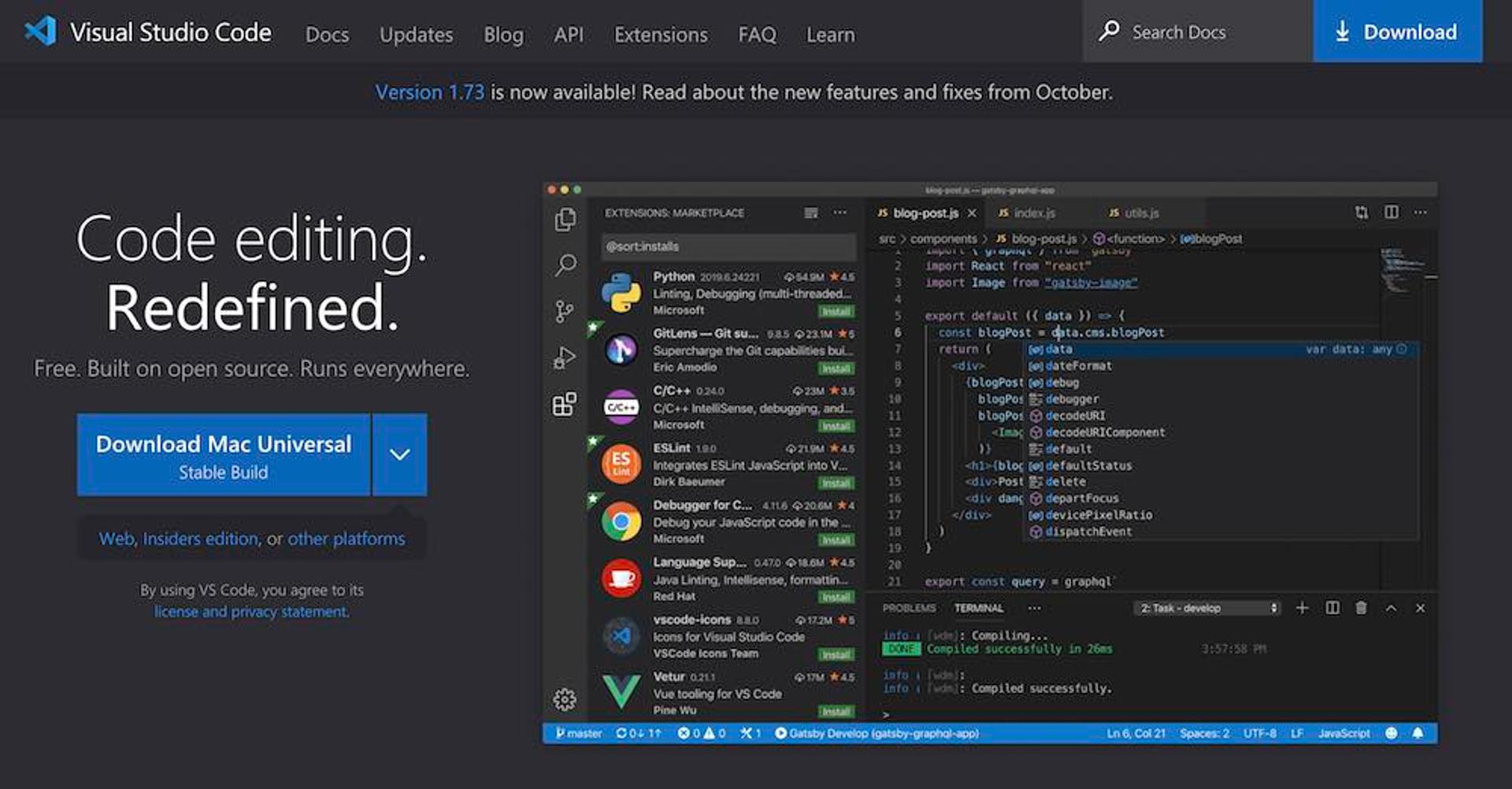
Pick up a framework
Many JavaScript developers use frameworks, as they add a ton of valuable features and simplify the development process. Choose one or two JavaScript frameworks to learn. If you want to work with a specific company, look up its job postings. Job descriptions usually list the frameworks they would like potential candidates to know.
Study the framework’s documentation and create a few test projects to get familiar with how they work.
Hone your skills with JS coding challenges
Coding requires problem-solving, and no two coding projects are the same. New coders often find this variation challenging. How do you practice for a wide variety of projects and needs?
You start building with JavaScript. Coding challenges help you build and apply your programming skills to real-life scenarios. For example, JavaScript30(opens new window) is a 30-day coding challenge. When you sign up, you’ll receive 30 JavaScript challenges each day.
Apply for a free tech apprenticeship program
If you want a more structured learning approach with hands-on experience, apply for Multiverse’s Software Engineering apprenticeship. You’ll learn JavaScript and other programming languages during the program.
Multiverse apprenticeships are not only completely free—you’ll also get to learn on the job with a full-time role and salary. That means you’ll get paid to learn code and other in-demand skills that can help you pursue a Software Engineer career path.
Apprentices can access one-on-one career coaching support, interview preparation, and more resources. You can begin the quick application process here.
Showcase your skills and build your portfolio website
Once you’re comfortable with your web development skills, you can show off your hard work by building your own website with JavaScript source code. You can make it from scratch and prove what you’ve learned to potential employers. Mix in case studies of your projects to show your progress and highlight your coding process.
There are no set rules for learning JavaScript, but consistency goes a long way. Take your time and keep practicing.